Golang Redis ile JWT Kimlik Doğrulaması
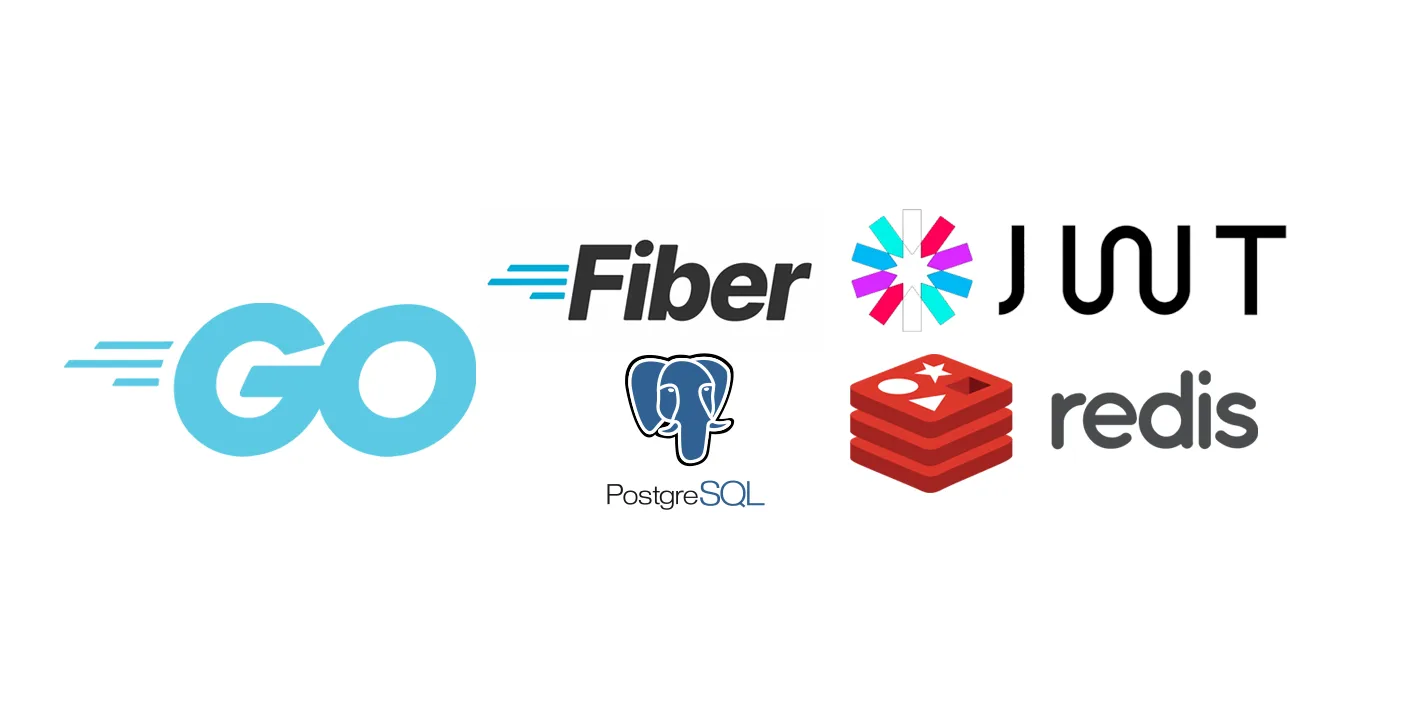
Golang Redis ile JWT Kimlik Doğrulaması
Bu makalede, Golang kullanarak JWT tabanlı bir kimlik doğrulama sistemi oluşturacağız. Sistemimiz şu teknolojileri kullanacak:
- Fiber: Hızlı bir web framework
- GORM: PostgreSQL ile etkileşim için ORM
- JWT: Kimlik doğrulama için JSON Web Token
- Redis: Token yönetimi için önbellek
- PostgreSQL: Kullanıcı verilerini depolamak için veritabanı
- Bcrypt: Şifre hashleme için güvenli algoritma
Yukarıda yazdıklarımız Golang Projesi içerisinde kullanacakalarımız bunlara ek olarak proje dışı bileşenler olarak
- Redis: Token yönetimi için önbellek
- PostgreSQL: Kullanıcı verilerini depolamak için veritabanı
Gereksinimler
Bu projeyi gerçekleştirmek için aşağıdaki araçlara ihtiyacınız olacak:
-
Go: En son sürümü https://golang.org/dl/ adresinden indirebilirsiniz.
-
PostgreSQL:
- İndirme: https://www.postgresql.org/download/
- IDE önerisi: pgAdmin (PostgreSQL ile birlikte gelir) veya DBeaver (https://dbeaver.io/)
PostgreSQL kurulumundan sonra:
- Yeni bir veritabanı oluşturun (örneğin:
auth_system
) - Kullanıcı adı, şifre ve veritabanı adını not edin (
.env
dosyasında kullanacağız)
-
Redis:
- İndirme: https://redis.io/download
- IDE önerisi: RedisInsight (https://redislabs.com/redis-enterprise/redis-insight/)
Redis kurulumundan sonra:
- Varsayılan olarak
localhost:6379
adresinde çalışacaktır - RedisInsight ile bağlanarak verileri görselleştirebilirsiniz
-
IDE:
- GoLand (https://www.jetbrains.com/go/) veya
- Visual Studio Code (https://code.visualstudio.com/) Go eklentisi ile
-
Postman: API’nizi test etmek için (https://www.postman.com/)
Proje Oluşturma
- Öncelikle, proje için yeni bir dizin oluşturun ve Go modülünü başlatın:
|
|
- Gerekli bağımlılıkları yükleyin:
|
|
Proje Yapısı
Projemizin yapısı şu şekilde olacak:
jwt-auth/ │ ├── config/ │ └── config.go ├── database/ │ └── database.go ├── handlers/ │ └── auth.go ├── middleware/ │ └── auth.go ├── models/ │ └── user.go ├── .env └── main.go
Env Dosyamız
- ‘.env’ dosyasını oluşturun ve aşağıdaki içerikleri kendi veritabanı bilgilerinizle doldurun:
|
|
Konfigürasyon
- ‘config’ dizini altında ‘config.go’ dosyasını oluşturun:
|
|
Veritabanı Bağlantısı
- ‘database’ dizini altında ‘database.go’ dosyasını oluşturun:
|
|
Modeller
- ‘models’ dizini altında ‘user.go’ dosyasını oluşturun:
|
|
Handler’lar
- ‘handlers’ dizini altında ‘auth.go’ dosyasını oluşturun:
|
|
Middleware
- ‘middleware’ dizini altında ‘auth.go’ dosyasını oluşturun:
|
|
Ana Dosya
- Son olarak, ‘main.go’ dosyasını oluşturun:
|
|
Uygulamayı Çalıştırma
- PostgreSQL ve Redis veritabanlarının çalıştığından emin olun.
- Ortamınızı ayarladıktan sonra, uygulamanızı şu komutla çalıştırabilirsiniz:
|
|
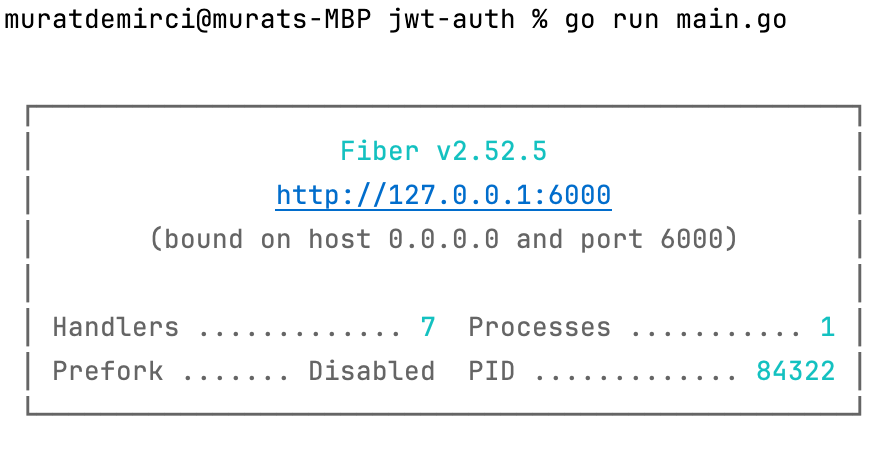
*Şekil 1: Uygulamanın çalışması *
Uygulamayı Test Etme
- Uygulamımızı ben postman Kullanarak test ettim sizde dilerseniz postman ile testlerinizi yapabilirsiniz.
Kullanıcı Oluşturma
- Postman ile post gönderimi yapacağız adres kısmına http://localhost:6000/register yazıyoruz ve Body kısmını raw seçip aşağıdaki json’ı yazıyoruz siz dilediğiniz kullanıcı adı ve şifreyle yapabilrisiniz
|
|
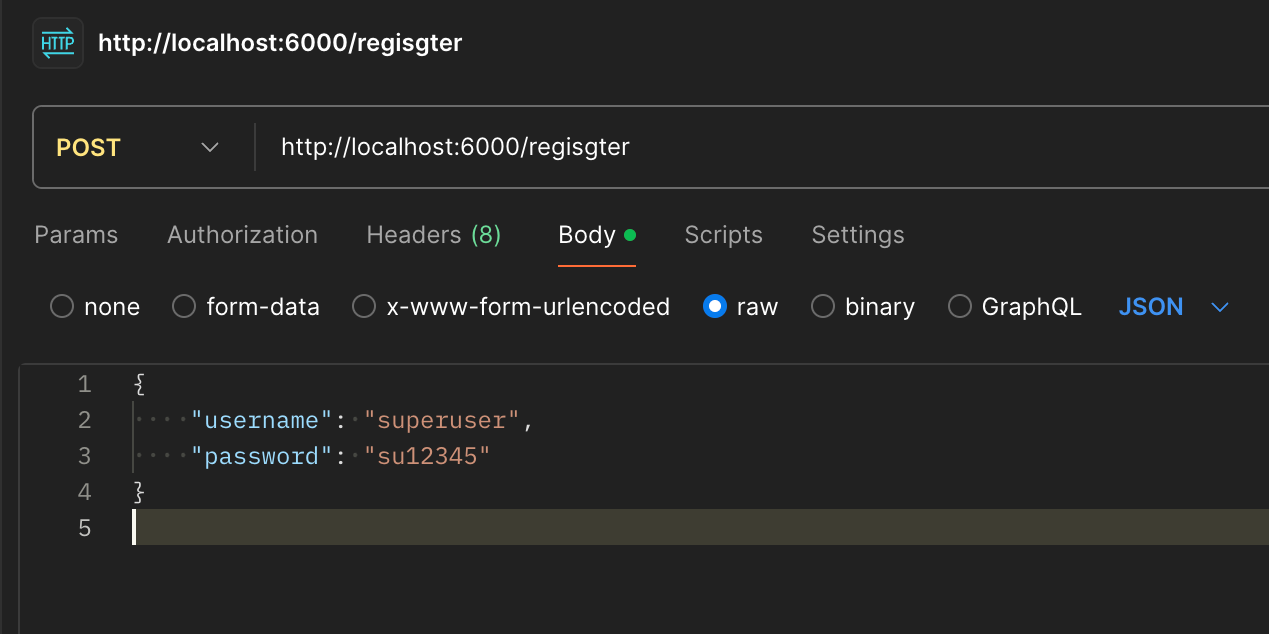
*Şekil 2: Postman Kullanıcı oluşturma*
- Postman ile gönderdiğimiz register post’una aldığımız cevap ta aşağıdaki gibi
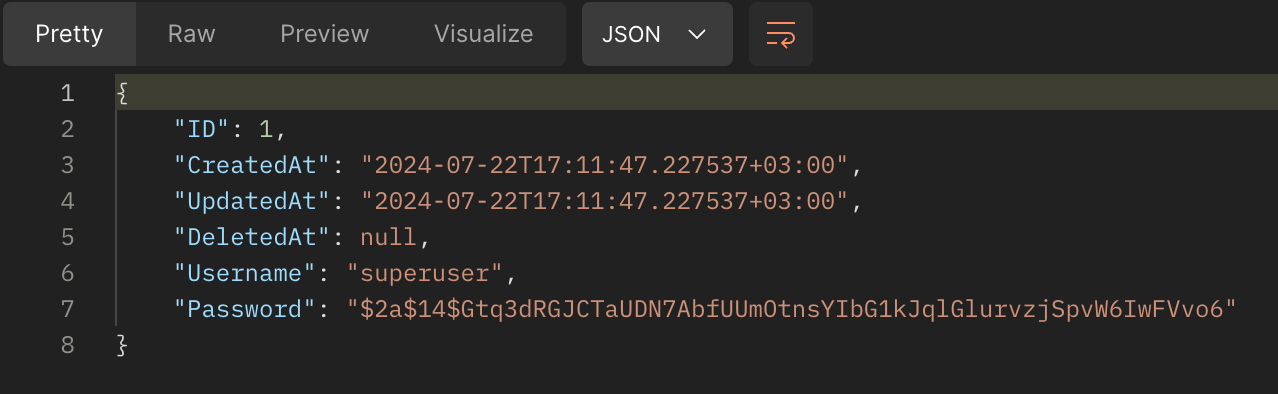
*Şekil 3: Postman Kullanıcı oluşturma Post Dönüşü *
- Veritabanında oluşan kayıt aşağıdaki gibi

*Şekil 4: Vertitabanın da oluşan kayıt *
Kullanıcı Girişi
- Postman ile post gönderimi yapacağız adres kısmına http://localhost:6000/login yazıyoruz ve Body kısmını raw seçip aşağıdaki json kısmına oluşturduğumuz kullanıcı adı ve şifreyi yazıyoruz.
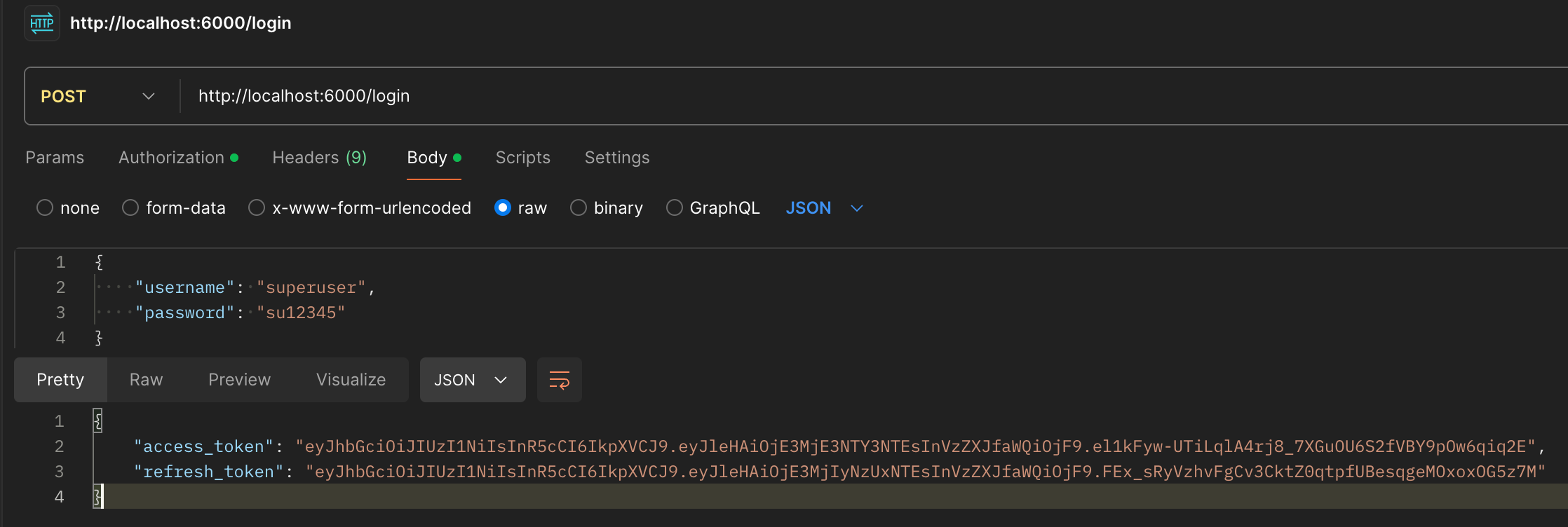
*Şekil 5: Postman İle Kullanıcı girişi *
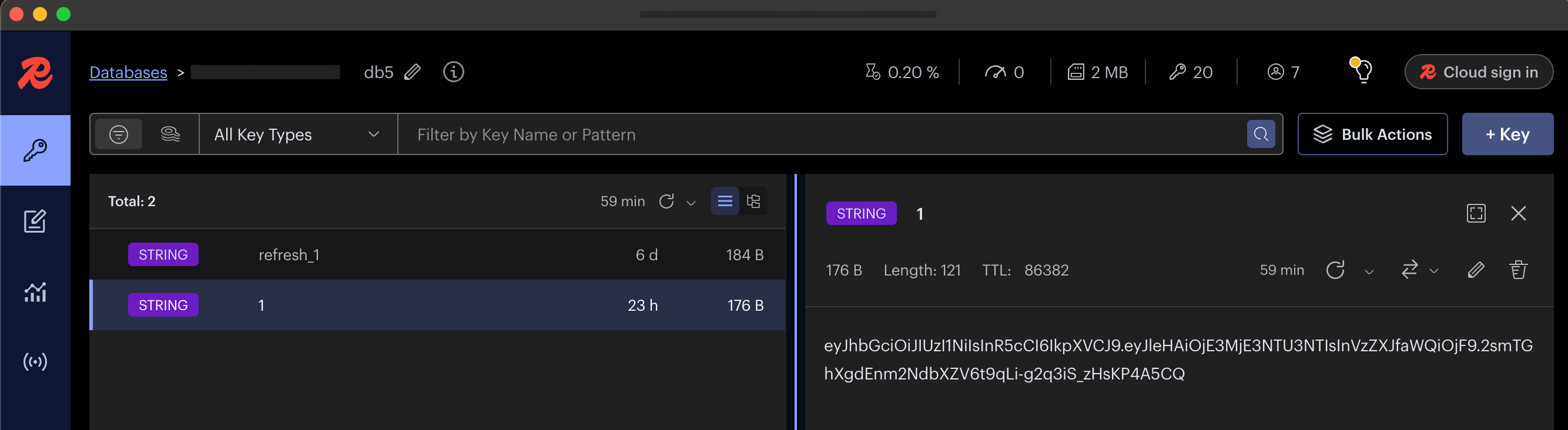
*Şekil 6: Rediste Tutlan token kayıtları *
Kullanıcı Detaylarını Alma
- Postman ile get işlemi yapacağız adres kısmına http://localhost:6000/user yazıyoruz ve Authentication kısmında Auth Type’ı Bearer Token seçip token kısmına not aldığım acces_token’ı yazıyoruz.
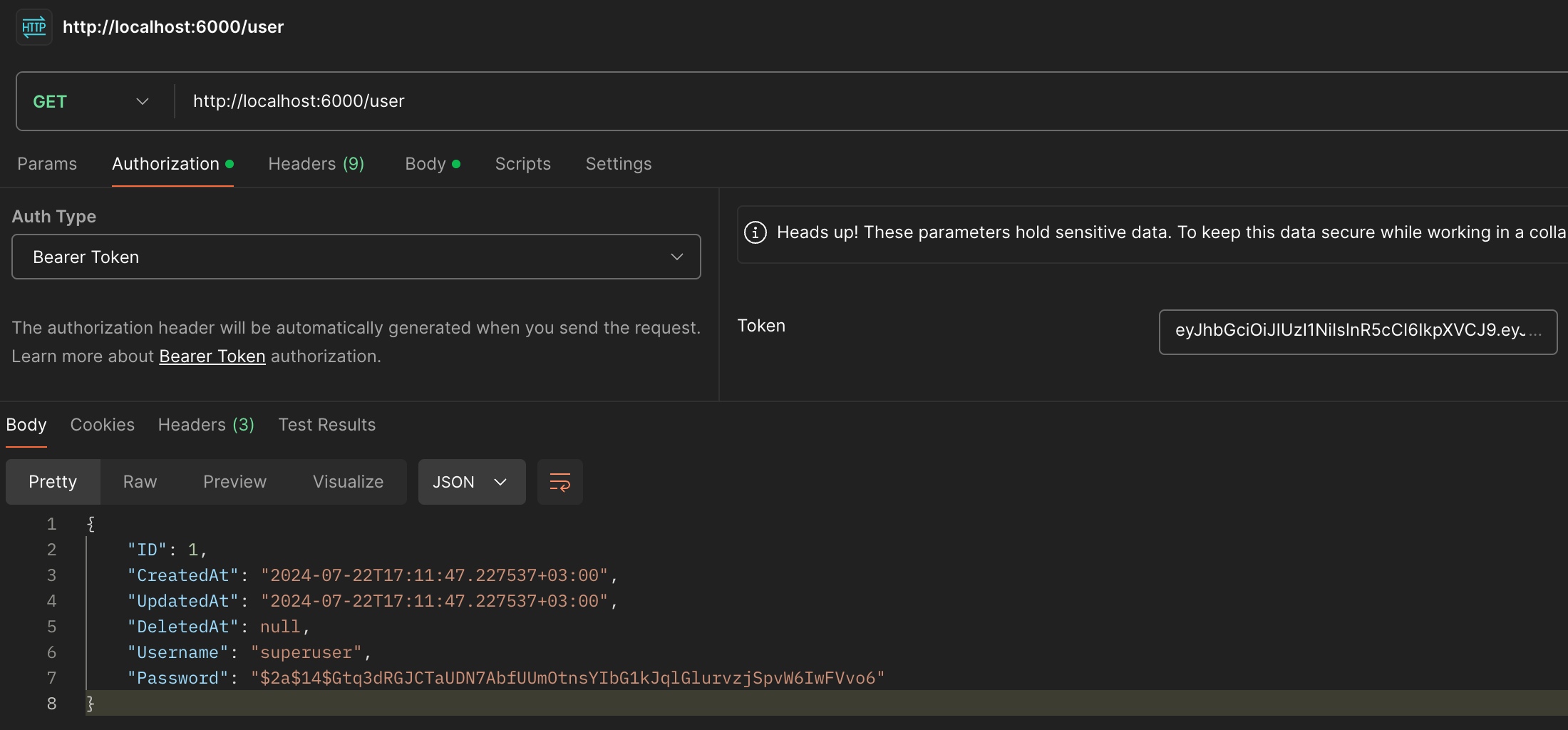
*Şekil 7: Kullanıcı Detayları *
Token Yenileme
- Postman ile post gönderimi yapacağız adres kısmına http://localhost:6000/user yazıyoruz başka birşey yapmadan send butonuna basabiliriz.
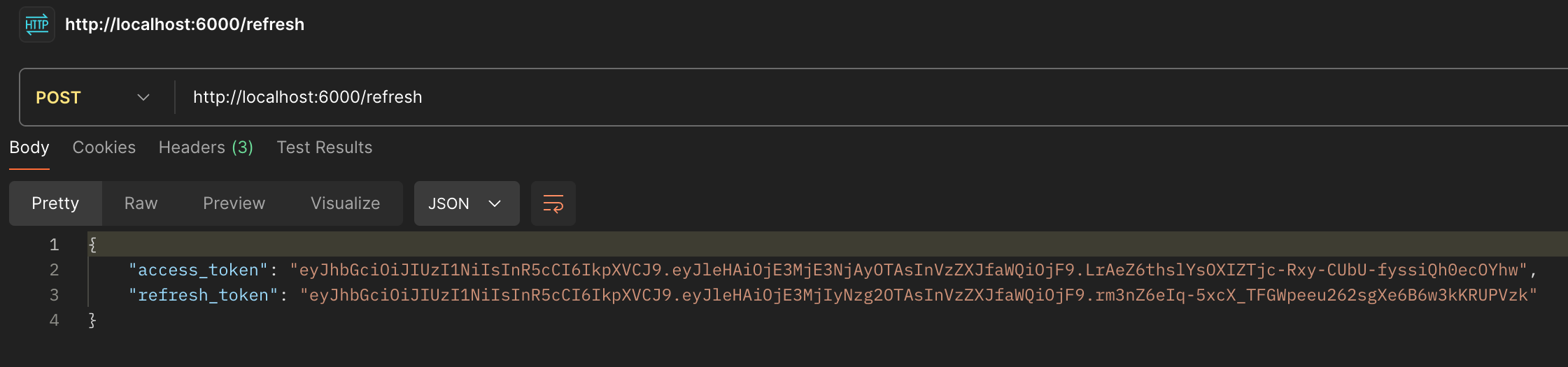
*Şekil 8: Token Yenileme *
Çıkış Yapma
- Postman ile post gönderimi yapacağız adres kısmına http://localhost:6000/logout yazıyoruz başka birşey yapmadan send butonuna basabiliriz.
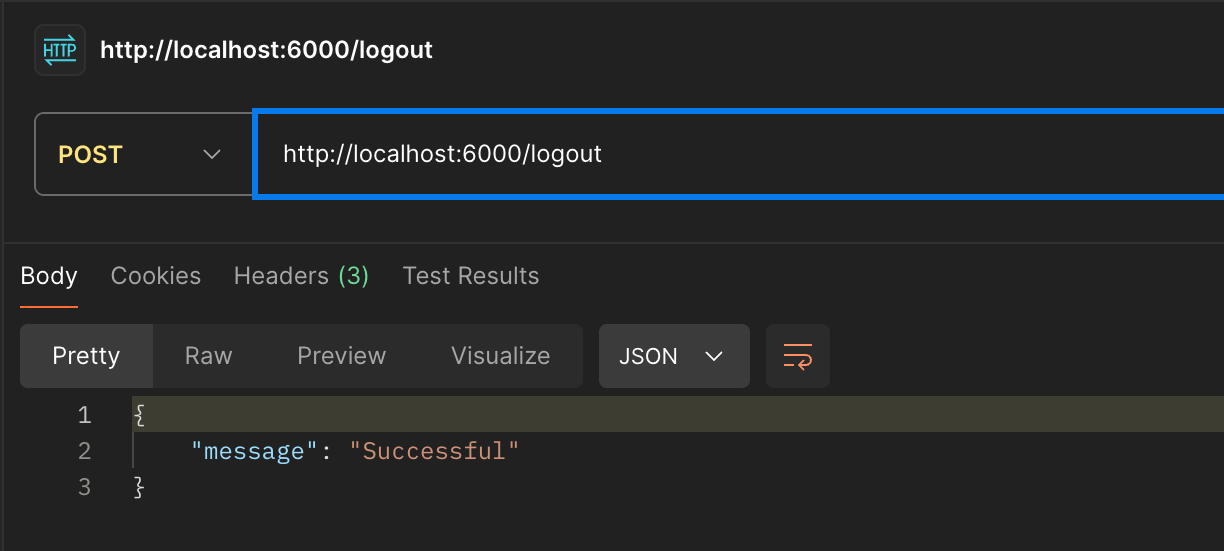
*Şekil 8: Çıkış yapma *
Sonuç
-
Bu makalede, Golang, Fiber, GORM, PostgreSQL ve Redis kullanarak JWT tabanlı bir kimlik doğrulama sistemi oluşturmayı adım adım anlatmaya çalıştım. Artık ihtiyacınıza göre ek özellikler ekleyebileceğiniz temel bir çerçeveye sahipsiniz.
-
Bu makalanin projelerinizi geliştirirken faydalı olmasını umuyorum. Sorularınız veya eklemelenmesi gereken noktalar varsa, yardımcı olmaktan memnuniyet duyarım!
-
Bir sonraki yazılarımda bu projenin Frontend kısmınıda Svelte kullanarak yazıp paylaşıyor olacağım.
-
Projenin kaynak kodlarına GitHub’dan ulaşabilirsiniz.Github