Mos Protocol Gateway With GOLANG
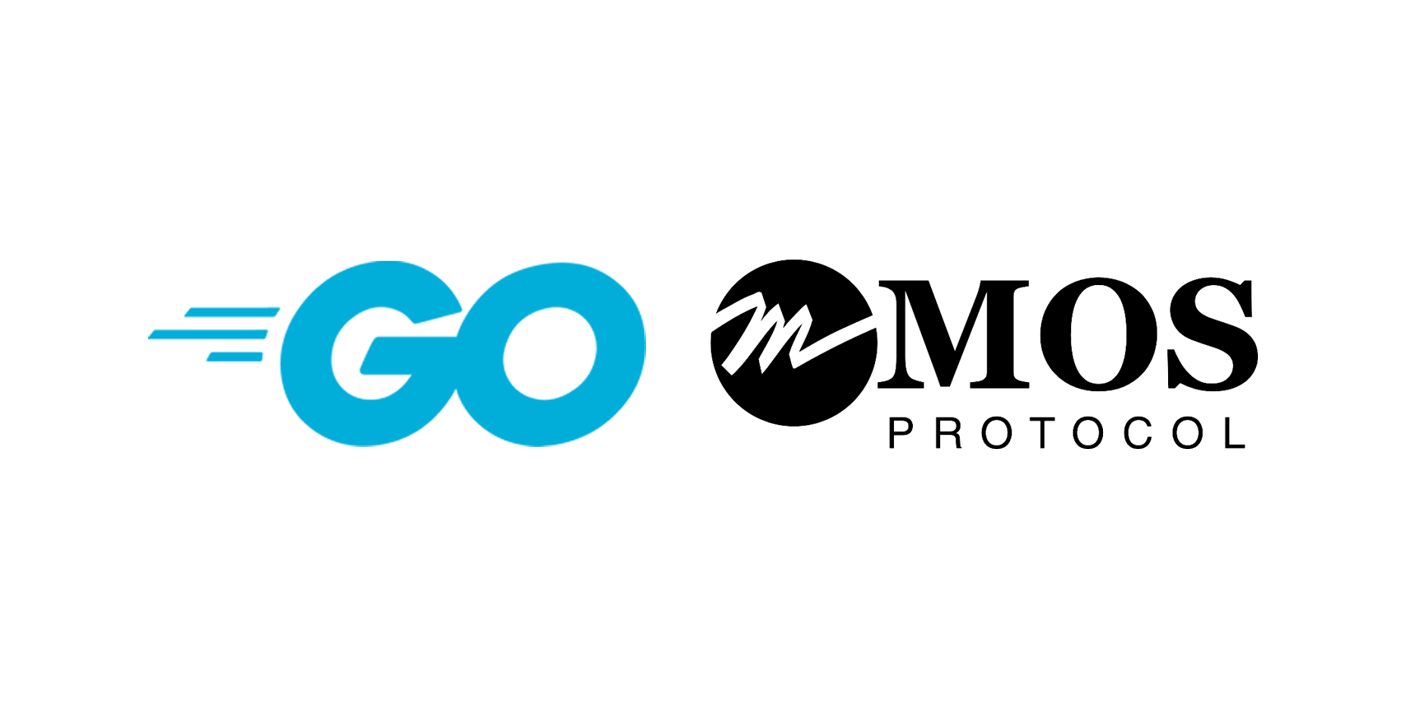
MOS (Media Object Server) Protocol: Technical Analysis and Implementation
Table of Contents
- Introduction
- Basic Structure of MOS Protocol
- MOS Protocol Implementation with Go
- Code Analysis and Examples
- Best Practices and Recommendations
1. Introduction
The MOS (Media Object Server) Protocol is a standardized protocol used for communication between systems in news broadcasting. This article examines the technical details of the protocol and its implementation using the Go programming language.
2. Basic Structure of MOS Protocol
MOS protocol uses an XML-based messaging system running over TCP/IP. The fundamental features of the protocol include:
- Dual port structure (10540 and 10541)
- XML format messaging
- UCS-2 character encoding
- Heartbeat mechanism
- Acknowledgment (ACK) system
3. MOS Protocol Implementation with Go
3.1. Basic Configuration
Configuration structure used for protocol implementation:
|
|
3.2. Heartbeat Mechanism
The heartbeat function that monitors the continuity of connections between systems:
|
|
3.3. Rundown Operations
Basic structures used for rundown management in MOS protocol:
|
|
3.4. Message Processing Mechanism
Main function used for processing incoming messages:
|
|
4. Important Implementation Details
4.1. XML Processing
XML processing is crucial in the MOS protocol. Structures are defined using Go’s xml package:
|
|
4.2. Logging System
Logging mechanism used for tracking system events:
|
|
4.3. ACK (Acknowledgment) Mechanism
ACK messages indicating successful operations:
|
|
5. Best Practices and Recommendations
5.1. Error Handling
- Use timeout mechanisms for every network operation
- Handle connection interruptions
- Implement detailed logging
5.2. Performance Optimization
- Optimize buffer sizes
- Avoid unnecessary XML parsing
- Implement connection pooling
5.3. Security Measures
- Validate all incoming data
- Consider SSL/TLS usage
- Implement access control
6. Conclusion
The MOS protocol implementation has a complex but well-organized structure. Go’s powerful concurrency features and XML processing capabilities enable efficient implementation of the protocol. The code examples and explanations above demonstrate the fundamental building blocks and implementation details of the protocol.
For a successful MOS implementation:
- Thoroughly understand the protocol specification
- Establish a robust error handling mechanism
- Implement detailed logging
- Consider performance optimizations
- Plan security measures from the start
This approach enables the implementation of a reliable and high-performance MOS protocol system.
7. Technical Specifications
7.1. Protocol Details
- Protocol Name: MOS (Media Object Server)
- Transport Layer: TCP/IP
- Ports: 10541 (Upper Port), 10540 (Lower Port)
- Message Format: XML
- Character Encoding: UCS-2
7.2. Key Components
- Heartbeat System
- Message Queue Management
- Rundown Operations
- Story Management
- Item Processing
- Metadata Handling
7.3. Message Types
- Basic Messages:
- Heartbeat
- ACK
- Error
- Rundown Operations:
- RoCreate
- RoDelete
- RoReplace
- RoList
- Story Operations:
- RoStoryAppend
- RoStoryDelete
- RoStoryMove
- RoStorySwap
8. Implementation Considerations
8.1. System Architecture
The implementation should consider:
- Scalability requirements
- High availability needs
- Network reliability
- Data persistence
- System monitoring
8.2. Development Guidelines
- Follow Go best practices
- Implement comprehensive error handling
- Use proper logging mechanisms
- Maintain clean code structure
- Document all critical components
8.3. Testing Strategy
-
Unit testing for individual components
-
Integration testing for message flows
-
Load testing for performance validation
-
Error scenario testing
-
Network failure recovery testing
-
You can access the complete source code of the project from this link Github Mos Protocol gateway with Golang